Example Introduction
Preparations
- Install Docker
- Install Git
- Install JDK 8.0
- Download Jenkins 2.8
- Download example
and run jenkins: nohup java -jar jenkins2.8.war &
git clone https://github.com/easynode/easynode-ipc.git
Example introduction
This example permits user to login system by SSO(URS), then storage the session of logined-user to a distributed K/V system(Redis), and allow users to fill out forms. after verification, the form data will be stored to RDS(likely mysql Server) and the form file will be stored to the NOS(net object storage) system. It provides some APIs of Restfule style for management backend to audit.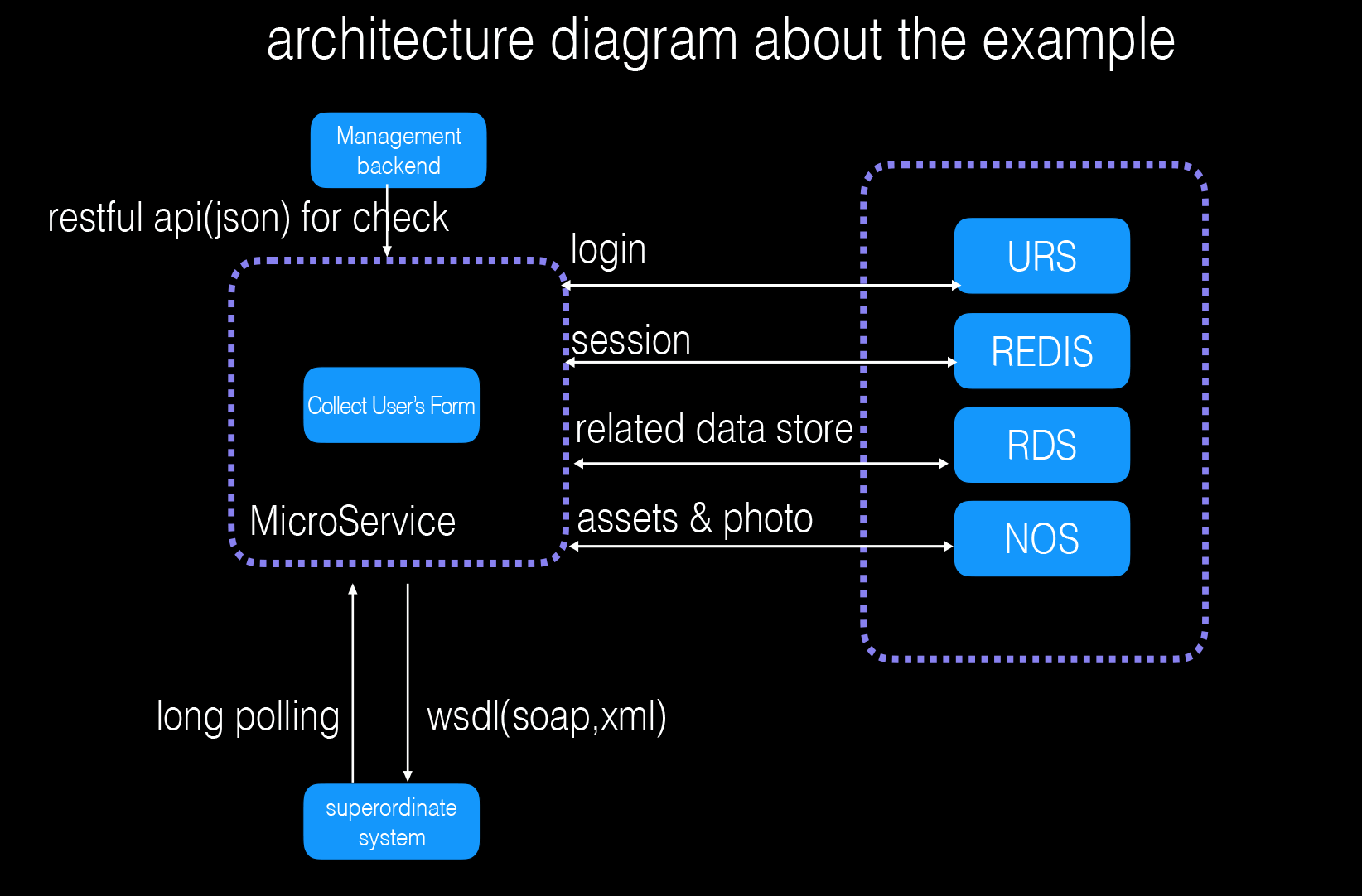
Directory structure of this example
.
├── bin
├── config
├── docs
├── etc
|── plugins
├── public
├── src
├── test
├── .babelrc
├── .dockerignore
├── Dockerfile
├── README.md
├── package.json
└── test.sh
The example directory:
File / Directory | Description |
---|---|
|
This directory stores the startup scripts and main programs for each environment (such as DEVELOP,TEST,PRODUCTION environment). Now you only need to care about the following two files: |
|
You can ignore this directory, the configuration will be provided by the configuration services |
|
You can save a number of documents related to the project, such as protocol documents, requirements design documents, meeting memories, etc. |
|
This is the internal configuration for the need of the Easynode framework You can ignore it now. |
|
The directory includes source code of a single page application based on react framework , and configuration for packing. see details |
|
This directory contains the source code of backend for this example. see details |
|
This directory contains the source code of test for main business lines |
|
The entire range of Babel API options are allowed. |
|
If a file named .dockerignore exists in the source repository, then it is interpreted as a newline-separated list of exclusion patterns. Exclusion patterns match files or directories relative to the source repository that will be excluded from the context. It can even be used to ignore the Dockerfile and .dockerignore files. |
|
Docker can build images automatically by reading the instructions from a Dockerfile. A Dockerfile is a text document that contains all the commands you would normally execute manually in order to build a Docker image. By calling docker build from your terminal, you can have Docker build your image step by step, executing the instructions successively. |
|
This file is the introduction of the project's portal, written in MarkDown format. |
|
Specifics of npm's package.json handling,It must be actual JSON, not just a JavaScript object literal. |
|
It just a test startup shell script, and can be run after the container has been started. |
File / Directory | Description |
---|---|
|
This directory contains the configuration and scripts to generate the API document of the backend's code. |
|
This directory stores a static resource file, such as a picture. |
|
This directory stores packaged files and mapped files for the front-end resource files such as html, css, js, png as suffix. |
|
An output directory of the MCSS tool. |
|
The front-end source code folder of the project, based on the React framework.
|
|
Third party dependence, will be replaced webpack. You can ignore it now. |
|
Modular css with mcss |
|
Static pages before design of components, You can ignore it now. |
|
Similar to the WWW directory, but can not be named as the WWW directory, because it has been occupied by the easynode framework |
|
Description document as a micro service interface. It contains the configuration and scripts to generate the Restful style API document |
|
This file is the introduction of the front-end's portal, written in MarkDown format. |
|
ESLint is designed to be completely configurable, meaning you can turn off every rule and run only with basic syntax validation, or mix and match the bundled rules and your custom rules to make ESLint perfect for your project. This is a configuration file tell eslint how to work. |
|
This is a configuration file tell mcss how to work. |
|
This is a configuration file tell webpack how to work for the development environment. |
|
This is a configuration file tell webpack how to work for the test or production environment. |
var LoginService = using('netease.icp.backend.services.LoginService');
var loginService = new LoginService(app);
File / Directory | Description |
---|---|
|
This is the controller layer of the MVC Pattern. Controller acts on both model and view. It controls the data flow into model object and updates the view whenever data changes. It keeps view and model separate. |
|
This is the model layer of the MVC Pattern, is not been used to render to views directly but carring data for protocol. |
|
This is the model layer of the MVC Pattern, is not been used to render to views directly but as a model of database. |
|
Routing refers to the definition of application end points (URIs) and how they respond to client requests. It's master responsiblity is to map the method and URIs and Generator. example:
|
|
This is the logic service layer of business. |
|
The main entrance of this program is similar to the main in Java or c/c++ language |
How to run the example
-
step 1:
Start a mysql container.
docker run --name mysql --restart=always -v `pwd`:/etc/mysql/conf.d -v `pwd`/db/:/var/lib/mysql -e MYSQL_ROOT_PASSWORD='test' -e MYSQL_DATABASE=test -e MYSQL_USER=test -e MYSQL_PASSWORD=test -p 3306:3306 -d mysql
-
step 2:
Start a redis container.
docker run -d --name redis -p 6379:6379 -v `pwd`/db/:/data redis redis-server /usr/local/etc/redis/redis.conf
-
step 3:
fill in the config.json , and you can store the file as the same position with dev_start.sh at bin directory
config.json If the config din't been encoded. You need to uncomment the line "config = JSON.parse(StringUtil.decryptAdv(config));" at the file Main.js. -
step 4:
build the image
docker build -t your_image_name .
-
step 5:
Run the example
docker run -ti --name dev_example -p 8888:8888 -v `pwd`:/usr/src/app --workdir=/usr/src/app/bin --env ENV=DEVELOP -e CONFIG_URL=/usr/src/app/bin/config.json -e PORT=8888 your_image_name ./start.sh
How to debug the example
-
step 1:
Run the container
docker run -ti --name dev_example -p 8888:8888 -v `pwd`:/usr/src/app --workdir=/usr/src/app/bin --env ENV=DEVELOP -e CONFIG_URL=/usr/src/app/bin/config.json -e PORT=8888 your_image_name /bin/bash
-
step 2:
Run the example
After entering the container, cd /usr/src/app/bin, and run
supervisor -w ../src -spid /var/tmp/icp.pid sh dev_start.sh
note: /var/temp/icp.pid is the value of the key easynode.app.id in the file etc/EasyNode.conf, The hot loader willn't work if you have not config the key.
-
step 3:
Debug the front-end code
cd plugins; webpack -w & mcss mcss/index.mcss -o css/ -w